Kling AI Image to Image
Model Information
Input
Configure model parameters
Output
View generated results
Result
Preview, share or download your results with a single click.
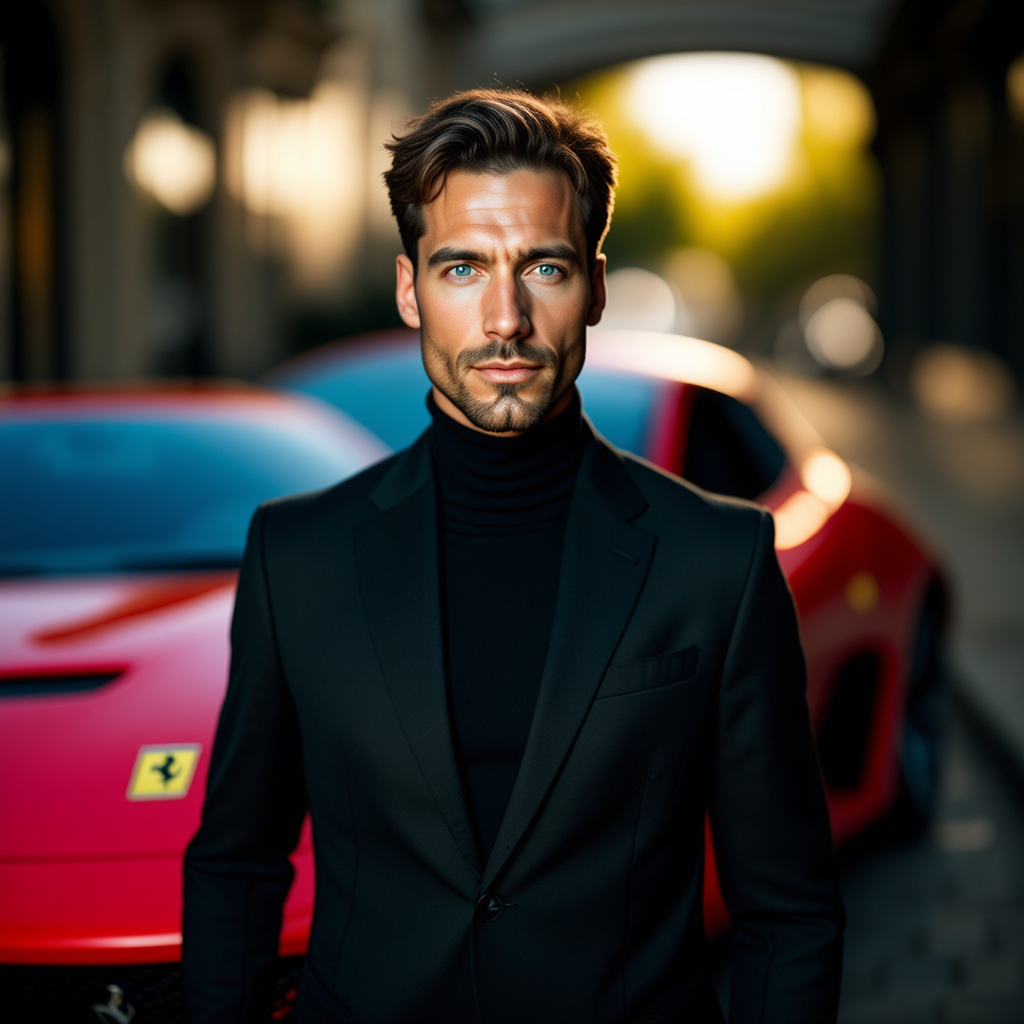
Prerequisites
- Create an API Key from the Eachlabs Console
- Install the required dependencies for your chosen language (e.g., requests for Python)
API Integration Steps
1. Create a Prediction
Send a POST request to create a new prediction. This will return a prediction ID that you'll use to check the result. The request should include your model inputs and API key.
import requestsimport timeAPI_KEY = "YOUR_API_KEY" # Replace with your API keyHEADERS = {"X-API-Key": API_KEY,"Content-Type": "application/json"}def create_prediction():response = requests.post("https://api.eachlabs.ai/v1/prediction/",headers=HEADERS,json={"model": "kling-v1-5-img-to-img","version": "0.0.1","input": {"aspect_ratio": "16:9","number_of_images": 1,"human_fidelity": 0.5,"image_fidelity": 0.5,"image_reference": "face","image": "your image here","prompt": "your prompt here","model": "kling-v1-5"},"webhook_url": ""})prediction = response.json()if prediction["status"] != "success":raise Exception(f"Prediction failed: {prediction}")return prediction["predictionID"]
2. Get Prediction Result
Poll the prediction endpoint with the prediction ID until the result is ready. The API uses long-polling, so you'll need to repeatedly check until you receive a success status.
def get_prediction(prediction_id):while True:result = requests.get(f"https://api.eachlabs.ai/v1/prediction/{prediction_id}",headers=HEADERS).json()if result["status"] == "success":return resultelif result["status"] == "error":raise Exception(f"Prediction failed: {result}")time.sleep(1) # Wait before polling again
3. Complete Example
Here's a complete example that puts it all together, including error handling and result processing. This shows how to create a prediction and wait for the result in a production environment.
try:# Create predictionprediction_id = create_prediction()print(f"Prediction created: {prediction_id}")# Get resultresult = get_prediction(prediction_id)print(f"Output URL: {result['output']}")print(f"Processing time: {result['metrics']['predict_time']}s")except Exception as e:print(f"Error: {e}")
Additional Information
- The API uses a two-step process: create prediction and poll for results
- Response time: ~50 seconds
- Rate limit: 60 requests/minute
- Concurrent requests: 10 maximum
- Use long-polling to check prediction status until completion
Overview
Kling AI Image to Image is a generative visual transformation model that takes a source image and modifies it according to a user-defined prompt. It is designed to preserve the structure and key features of the original image while creatively adapting stylistic and semantic elements. Kling AI Image to Image enables detailed and artistic reinterpretations of existing visuals, merging user intent with visual context in a controlled manner.
Technical Specifications
Kling AI Image to Image incorporates dual fidelity paths: one for structure (image fidelity) and one for facial/human elements (human fidelity).
High-resolution processing is supported with aspect ratio flexibility.
Kling AI Image to Image is optimized for stable output consistency and sharp visual detail, with prompt adherence being a key design priority.
Ensure the uploaded image is clear and well-lit; low-quality or blurry images may reduce visual fidelity.
Prompts should be descriptive and specific to guide Kling AI Image to Image effectively (e.g., "cyberpunk street at night" instead of just "street").
Use a face or subject reference only when strong identity preservation is needed. Avoid using both at once unless required.
Fidelity sliders allow for fine-grained control over how much the original image or human features are preserved.
Use the aspect ratio that aligns with your final medium or purpose (e.g., 1:1 for avatars, 9:16 for vertical posters).
Key Considerations
Outputs are directly influenced by the quality and framing of the input image.
Extremely abstract or unrelated prompts may reduce image consistency.
Aspect ratios outside the standard range may result in stretching or cropping.
When using facial references, ensure alignment and clarity for accurate facial preservation.
Repetitive prompts or overly generic instructions may result in outputs that feel less refined or generic.
Legal Information for Kling AI Image to Image
By using this Kling AI Image to Image, you agree to:
- Kling Privacy
- Kling SERVICE AGREEMENT
Tips & Tricks
To get the most consistent and visually rich outputs from Kling AI Image to Image:
- Prompt: Use detailed phrases. Combine style, subject, and environment (e.g., "futuristic knight with glowing armor in misty forest").
- Image: Start with an image that clearly shows the subject in proper lighting. Avoid backgrounds that are overly cluttered.
-
Image Reference:
- Use "face" if preserving facial features is your priority (e.g., stylized portraits).
- Use "subject" for keeping the pose, silhouette, or object layout consistent.
-
Image Fidelity:
- A value close to 1.0 means more of the original image is retained.
- A value close to 0.0 allows more freedom for Kling AI Image to Image to creatively reinterpret.
- Suggested range: 0.6 – 0.8 for balanced transformation.
-
Human Fidelity:
- Set closer to 1.0 to preserve recognizable human features (good for portraits).
- Use 0.3 – 0.5 if you want stylized or non-human transformation.
-
Aspect Ratio:
- Use 16:9 for widescreen visuals.
- Use 1:1 for avatars or profile-style images.
- Use 9:16 for vertical mobile display content.
Capabilities
Transforms existing images with prompt-guided modifications.
Preserves structure and composition while changing theme, style, and context.
Offers high control over human likeness and structural retention.
Supports multiple output formats and aspect ratios.
Enables style transfer for portraits, character art, and thematic reinterpretations.
What can I use for?
Creating stylized portraits from real photos.
Turning product images into themed promotional visuals.
Enhancing concept art with descriptive prompts.
Creating social media assets with consistent subjects and artistic effects.
Visualizing character transformations in different settings or eras.
Generating promotional or cinematic artwork from basic references.
Things to be aware of
Upload a standard headshot and use prompts like "regal queen with golden crown and flowing robe" to explore character design.
Use "subject" image reference and prompt "anime-style night warrior with electric sword" for stylized action scenes.
Set image fidelity to 0.7 and human fidelity to 0.9 for semi-realistic portrait enhancement.
Explore different aspect ratios to match platform requirements — vertical for stories, square for avatars, wide for banners.
Limitations
Not ideal for heavily abstract or surreal prompts that conflict with the input image structure.
Human anatomy preservation may weaken at low human fidelity values.
Overly artistic outputs may lose fine details of the original image.
Non-standard input image sizes or formats may impact output alignment.
Repeated generation with identical parameters may still produce slight variations due to model randomness.
Output Format: PNG