Input
Configure model parameters
Output
View generated results
Result
Preview, share or download your results with a single click.
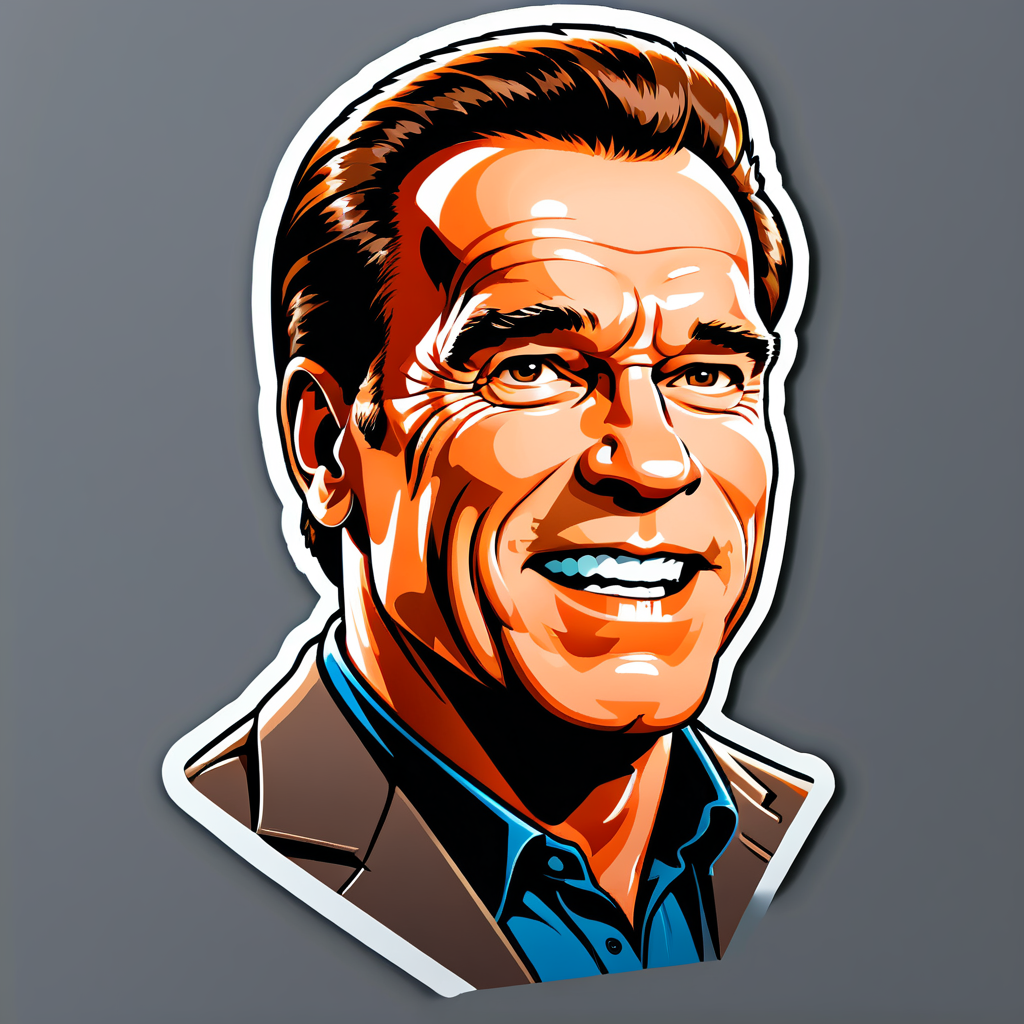
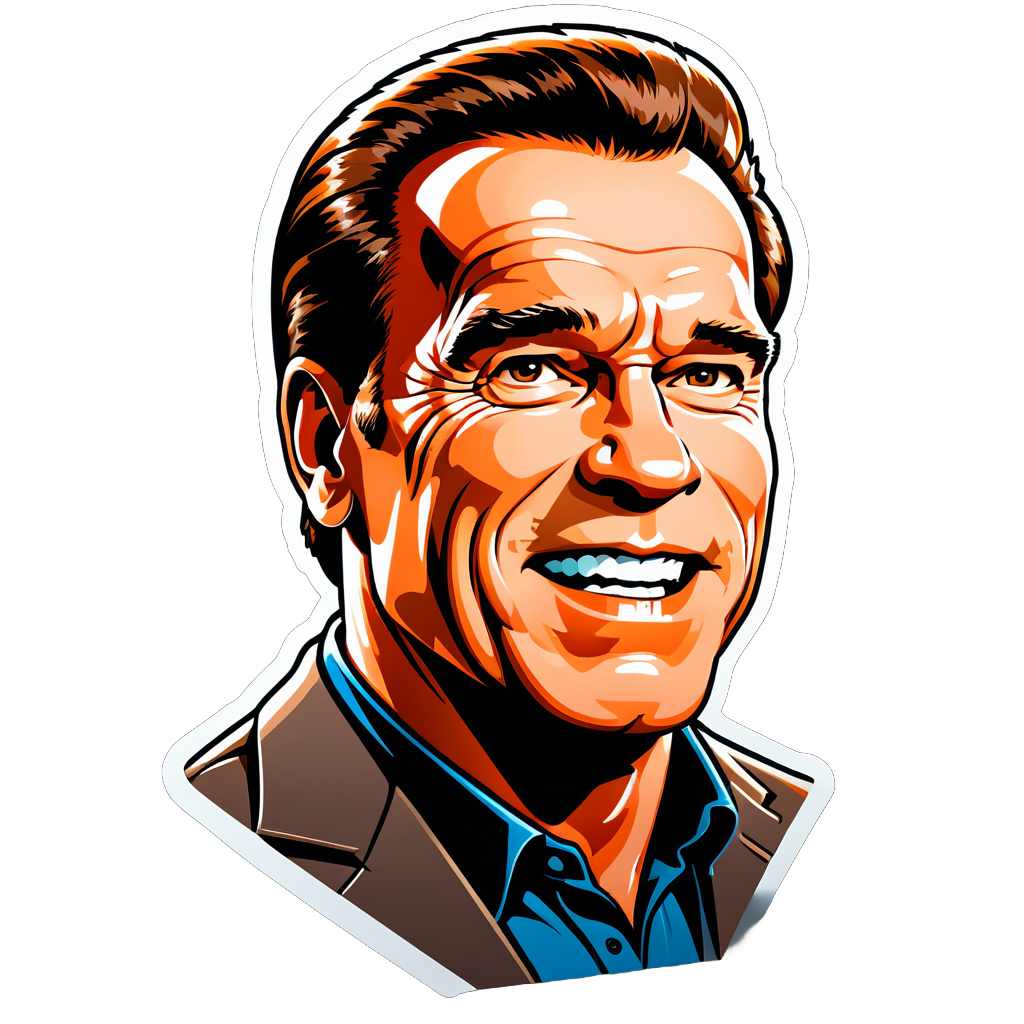
Prerequisites
- Create an API Key from the Eachlabs Console
- Install the required dependencies for your chosen language (e.g., requests for Python)
API Integration Steps
1. Create a Prediction
Send a POST request to create a new prediction. This will return a prediction ID that you'll use to check the result. The request should include your model inputs and API key.
import requestsimport timeAPI_KEY = "YOUR_API_KEY" # Replace with your API keyHEADERS = {"X-API-Key": API_KEY,"Content-Type": "application/json"}def create_prediction():response = requests.post("https://api.eachlabs.ai/v1/prediction/",headers=HEADERS,json={"model": "face-to-sticker","version": "0.0.1","input": {"seed": 0,"image": "your_file.image/jpeg","steps": 20,"width": 1024,"height": 1024,"prompt": "a person","upscale": false,"upscale_steps": 10,"negative_prompt": "your negative prompt here","prompt_strength": 7,"ip_adapter_noise": 0.5,"ip_adapter_weight": 0.2,"instant_id_strength": 1},"webhook_url": ""})prediction = response.json()if prediction["status"] != "success":raise Exception(f"Prediction failed: {prediction}")return prediction["predictionID"]
2. Get Prediction Result
Poll the prediction endpoint with the prediction ID until the result is ready. The API uses long-polling, so you'll need to repeatedly check until you receive a success status.
def get_prediction(prediction_id):while True:result = requests.get(f"https://api.eachlabs.ai/v1/prediction/{prediction_id}",headers=HEADERS).json()if result["status"] == "success":return resultelif result["status"] == "error":raise Exception(f"Prediction failed: {result}")time.sleep(1) # Wait before polling again
3. Complete Example
Here's a complete example that puts it all together, including error handling and result processing. This shows how to create a prediction and wait for the result in a production environment.
try:# Create predictionprediction_id = create_prediction()print(f"Prediction created: {prediction_id}")# Get resultresult = get_prediction(prediction_id)print(f"Output URL: {result['output']}")print(f"Processing time: {result['metrics']['predict_time']}s")except Exception as e:print(f"Error: {e}")
Additional Information
- The API uses a two-step process: create prediction and poll for results
- Response time: ~34 seconds
- Rate limit: 60 requests/minute
- Concurrent requests: 10 maximum
- Use long-polling to check prediction status until completion
Overview
The Face to Sticker model transforms facial images into creative, sticker-like representations. It allows users to generate unique and personalized sticker-style outputs using advanced image processing and transformation techniques. Face to Sticker model is versatile and efficient, providing control over various parameters to customize the final result according to user preferences.
Technical Specifications
Model Purpose: Converts facial images into stylized stickers using advanced deep learning techniques.
Customizable Outputs: Multiple settings such as Prompt Strength, Steps, and Upscale Steps provide users with flexibility and control.
Key Considerations
Input Image Quality: The model performs best when the input image is sharp and properly framed. Avoid blurry or low-resolution images.
Output Resolution: Larger Width and Height values result in higher-quality stickers but may increase processing time.
Resource Efficiency: Using fewer Steps can speed up processing but may slightly reduce output quality.
Tips & Tricks
Input Settings for Face to Sticker
- Image: Use high-resolution images (minimum 512x512 pixels) with a centered face to achieve the best results.
- Prompt: Use clear and specific prompts such as "cartoon-style sticker with vibrant colors" to guide the model's output effectively.
- Negative Prompt: Define undesirable features (e.g., "blurred edges") to avoid them in the output.
Adjustable Parameters for Face to Sticker
- Width and Height: Keep these values between 512 and 1024 for a balance between quality and speed. Larger values (e.g., 1024x1024) yield more detailed stickers but require more processing power.
- Steps: A range of 20-50 steps is recommended for high-quality outputs. Higher values may improve details but can increase processing time.
- Seed: Use a fixed seed to reproduce specific results consistently. Randomize the seed for unique variations.
- Prompt Strength: A value between 0.5 and 0.8 ensures the model respects the prompt while retaining facial details.
- Instant ID Strength: Values close to 1 prioritize facial identity, while lower values focus more on stylization. A typical range is 0.6 to 0.9.
- IP Adapter Weight: For subtle adjustments, values between 0.2 and 0.8 are optimal.
- IP Adapter Noise: Set between 0.1 and 0.5 to introduce controlled variations without compromising quality.
- Upscale: Enable this feature when generating final outputs to enhance resolution and clarity. Recommended Upscale Steps range from 50 to 100 for best results.
Capabilities
Stylized Sticker Creation: Converts facial images into customized stickers in a variety of styles.
Customizable Outputs: Allows fine-tuning of multiple parameters for personalized results.
High-Quality Outputs: Delivers crisp and detailed stickers with minimal artifacts.
What can I use for?
- Personalization: Create unique stickers for personal use or gifting.
- Social Media Content: Enhance online presence with custom, stylized graphics.
- Merchandising: Develop stickers for commercial purposes, such as branding or product packaging.
Things to be aware of
Test different prompts and Prompt Strength values to explore creative variations.
Adjust IP Adapter Weight and Noise for subtle or dramatic effects.
Use the Upscale feature to generate high-quality stickers suitable for printing.
Limitations
- May not perform well with group photos or images with multiple faces.
- Overly complex prompts can lead to inconsistent results.
- High-resolution outputs might take longer to process depending on the selected parameters.
Output Format: PNG
Related AI Models
You can seamlessly integrate advanced AI capabilities into your applications without the hassle of managing complex infrastructure.